SpringBoot开发,通过JPA如何做数据库增删改查?
发布于 作者:苏南大叔 来源:程序如此灵动~

SpringBoot
和数据库交互的技术规范叫做JPA
: Java Persistence API
,具体实现这一思想的框架叫做Hibernate
。本文以user
表为例,演示对数据库的增删改查操作。值的一提的是:mybatis
是另外的数据库交互框架,和本文无关。
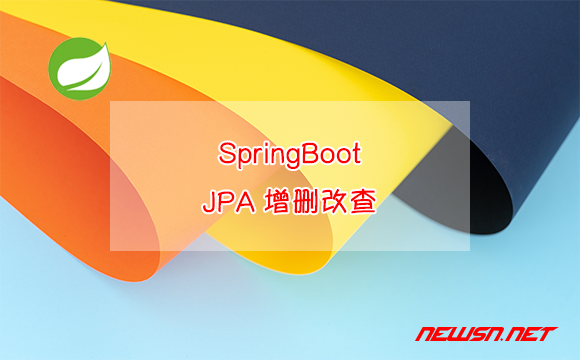
苏南大叔的“程序如此灵动”博客,记录苏南大叔的代码编程经验总结。测试环境:win10
,openjdk@23.0.2
,IntelliJ IDEA 2024.3.4.1
,maven@3.3.2
,spring boot@2.5.4
,java@17
,mysql@5.7.26
。
前文回顾
前文中,通过对user
表的数据查询,实现了用户登陆的功能。使用的代码包括model
(entity
)+controller
。参考文章:
在此基础上,通过session
来固化上面的查询结果,作为当前用户的身份凭证:
本文中,在原有的entity
和controller
的概念基础上,增加了repository
和service
的概念。那么,如何理解这四种概念的分工呢?
概念区分
在Spring Boot
中,Entity
、Controller
、Repository
和Service
四种组件各自扮演不同的角色,共同构建【微服务】架构:
Entity
:定义数据库模型,代表现实世界的实体,如订单、商品等,使用@Entity
注解。相当于把数据表用代码的方式再解释一遍。Controller
:负责业务逻辑,处理HTTP
请求,如用户登录、商品详情等。使用@RestController
或@Controller
注解。是直接和用户打交道的部分,对外提供地址访问的功能。Repository
:数据库操作的接口,执行增删改查操作。使用@Repository
进行注解,值得注意的是:一部分findById()
之类的操作是内置的,并不需要显式的写出来。Service
:业务逻辑和服务实现,处理事务,如服务工厂。使用@Service
,负责业务逻辑实现,不直接与数据库交互。或者说是在Repository
基础上的再包装一些业务逻辑。
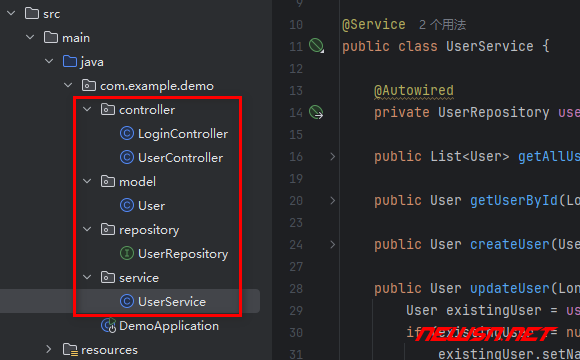
数据表user
JPA依赖及配置
pom.xml
:
然后就执行mvn install
命令。
resources/application.properties
新增配置:
entity及controller
src/main/java/com/example/demo/model/user.java
:
src/main/java/com/example/demo/controller/UserController.java
:
在这个例子里面,RestFul
的思想,表现的淋漓尽致啊。参考文章:
repository及service
src\main\java\com\example\demo\repository\UserRepository.java
:
对于这个文件里面的方法,可以看到。目前仅有一个存在,但是事实上,有很多方法隐藏了起来。参考文献:
src\main\java\com\example\demo\service\UserService.java
:
在Repository
基础上的在增加外置业务逻辑就是Service
。
template
这个模版是在上次登陆成功页面的基础上做的,所以,要访问的是定义在LoginController
里面的/success
页面。
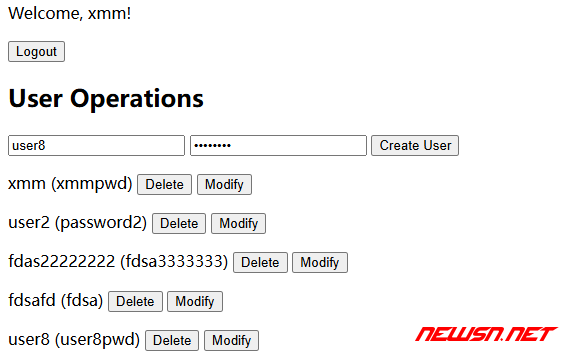
src\main\resources\templates\success.html
:
结语
遗留的比较大的问题是:这些接口的调用是在登陆成功的/success
,但是但就接口本身来说,它并没有做权限验证。那么在后续的文章里面,再讨论这个RestFul
接口的权限验证问题。
更多苏南大叔的java
经验文字,请点击:


